I’ve found myself writing a lot of import module statements during React development that I could have shortened. It’s a bit dispiriting when everything above the fold in your code is a boilerplate import. To give an example – this very simple React page
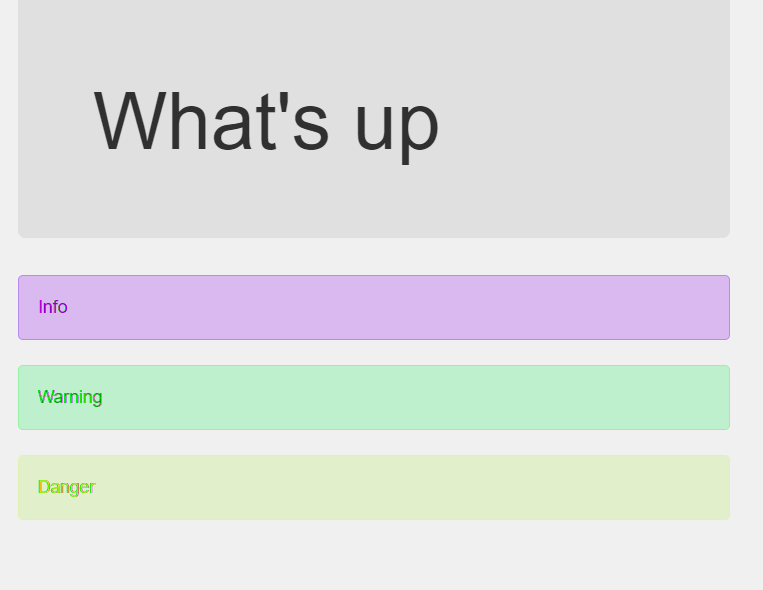
Is constructed from this component
import React from 'react'; var Info = require('./Labels.jsx').Info; var Warning = require('./Labels.jsx').Warning; var Danger = require('./Labels.jsx').Danger; export default class Page1 extends React.Component { render() { return <div> <div className="jumbotron"> <h1>What's up</h1> </div> <Info /> <Warning /> <Danger /> </div>; } }
Even in this simple example, I’ve a bunch of imports from the same Alerts module. This could potentially go on and on and has done when I’m using something like React-Bootstrap. It’s not pretty. Happily this can all be shortened to one line thus
var {Info, Warning, Danger} = require('./Labels.jsx')
When I first saw this I found it baffling and unexplained – especially when just importing 1 object from a module e.g.
var {Info} = require('./Labels.jsx')
Suddenly there is curly brackets in the way. What’s all that about?
It’s all in the Destructure
It’s using ES6 object destructuring to do this magic. A simple example of object destructuring would be
//.. the object var o = {p: 42, q: true}; //.. destructuring it into p and q var {p, q} = o; // p = 42 // q = true
The first object is automatically split into 2 variable when assigned – this is the destructuring
So our react import is the same as this in essence. Label.jsx is the component (object) we are importing
import React from 'react'; var Info = class extends React.Component { render() { return <div className="alert alert-info">Info</div>; } } var Warning = class extends React.Component { render() { return <div className="alert alert-warning">Warning</div>; } } var Danger = class extends React.Component { render() { return <div className="alert alert-danger">Danger</div>;; } } module.exports = { Info: Info, Warning: Warning, Danger: Danger }
The export at the end is what is going to be destructured – we could simplify this further and omit the property names (with more ES6)
module.exports = { Info, Warning, Danger }
Anyway that’s not strictly relevant. Explaining onwards – the require squirts out the object and the destructuring assigns it to 3 variables which we can use in our page
var {Info, Warning, Danger} = require('./Labels.jsx')
This can be rewritten as an ES6 import statement which will transpile down into require with something like Babel if need be.
import {Info, Warning, Danger} from './Labels.jsx'
It’s this form in particular I often see in code samples and the like. So that’s explained then and a little bit of the grey fog has lifted. Lovely.
Useful Links
https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Operators/Destructuring_assignment
ES6 Destructuring – good detailed explanation
https://blog.risingstack.com/node-js-at-scale-module-system-commonjs-require/
This is all underpinned by Node.js CommonJS module load pattern – that’s the require bit. More detail above.
https://github.com/timbrownls20/Demo/tree/master/React/CommonJSDemo
The sample code isn’t much but as ever the code is on my GitHub site. It’s using webpack as the task runner which is different to the gulp runner I’ve been using. Probably better if I’m honest.