I’ve written a few posts about Docker now so I thought I would just step back and write a set of instructions on how to browse the file system via an interactive shell on a running container. Although it’s basic I’d like to just reference these kind of instructions in other posts so I can avoid repeating myself. Also, people need simple guides to basic processes anyway – just watch me with a powerdrill and you’ll see someone in dire need of a basic guide.
Environment
I’m running Docker on a windows machine but I’ll be bring up windows and Linux containers.
The test project is a simple .Net Core project for managing student tests which I’ve ambitiously called Learning Analytics.
Windows Container
Using this simple DockerFile
FROM mcr.microsoft.com/dotnet/core/aspnet:3.1-nanoserver-1903 AS base WORKDIR /app EXPOSE 80 EXPOSE 443 FROM mcr.microsoft.com/dotnet/core/sdk:3.1-nanoserver-1903 AS build WORKDIR /src COPY ["LearningAnalytics.API/LearningAnalytics.API.csproj", "LearningAnalytics.API/"] RUN dotnet restore "LearningAnalytics.API/LearningAnalytics.API.csproj" COPY . . WORKDIR "/src/LearningAnalytics.API" RUN dotnet build "LearningAnalytics.API.csproj" -c Release -o /app/build FROM build AS publish RUN dotnet publish "LearningAnalytics.API.csproj" -c Release -o /app/publish FROM base AS final WORKDIR /app COPY --from=publish /app/publish . ENTRYPOINT ["dotnet", "LearningAnalytics.API.dll"]
Build it into an image
docker build . -f "LearningAnalytics.API\DockerFile" -t learninganalyticsapi:dev
It will be named learninganalyticsapi and tagged dev.
Now run the image as a container called learninganalyticsapi_app_1 in detached mode.
docker run -d -p 80:80 --name learninganalyticsapi_app_1 learninganalyticsapi:dev dotnet c:/app/publish/LearningAnalytics.API.dll
It’s going to bind the output of the api to port 80 of the host. Assuming there is nothing already bound to port 80, I can navigate to a test page here
http://localhost/test
And I will get a test message which confirms the container is up and running.
Now run the cmd shell in inteactive mode
docker exec -it learninganalyticsapi_app_1 cmd
Now we are on the running container itself so running these commands
cd .. dir
will navigate up to the root of the container and I can see what the top level directories are like so ….
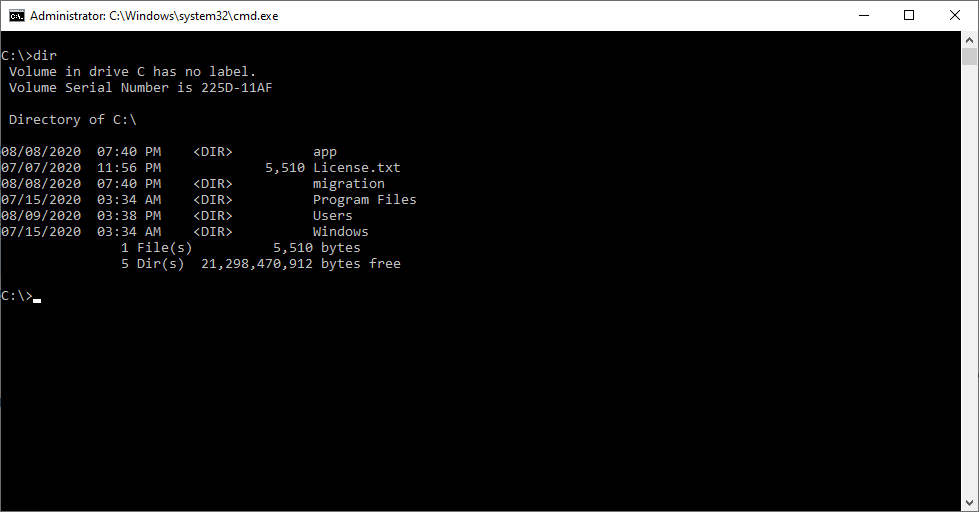
Obviously now I’ve got an interactive shell I can do anything that shell supports. Browsing files is just an easy example.
Once I’m done then type exit to end the interactive session and I’m back to the host.
Linux Container
So same again for a Linux container. It’s going to be pretty similar
Using this simple Docker file
FROM mcr.microsoft.com/dotnet/core/aspnet:3.1-buster-slim AS base WORKDIR /app EXPOSE 80 EXPOSE 443 FROM mcr.microsoft.com/dotnet/core/sdk:3.1-buster AS build WORKDIR /src COPY ["LearningAnalytics.API/LearningAnalytics.API.csproj", "LearningAnalytics.API/"] RUN dotnet restore "LearningAnalytics.API/LearningAnalytics.API.csproj" COPY . . WORKDIR "/src/LearningAnalytics.API" RUN dotnet build "LearningAnalytics.API.csproj" -c Release -o /app/build FROM build AS publish RUN dotnet publish "LearningAnalytics.API.csproj" -c Release -o /app/publish FROM base AS final WORKDIR /app COPY --from=publish /app/publish . ENTRYPOINT ["dotnet", "LearningAnalytics.API.dll"]
Build and run the container
docker build . -f "LearningAnalytics.API\DockerFile" -t learninganalyticsapi:dev docker run -d -p 49501:80 --name learninganalyticsapi_app_2 learninganalyticsapi:dev dotnet c:/app/publish/LearningAnalytics.API.dll
The only difference here is that I’ve bound it to a different port on the host.I’m working against port 49501. It’s just because I’ve already bound to port 80 in the first example so it’s now in use. If I use port 80 again then I get these kind of errors. So the test page for the Linux box is at
http://localhost:49501/test
Also the name of the container is learninganalyticsapi_app_2 to differentiate it from the Windows one which is already there from the first example.
Now bring up the shell, which is bash for Linux
docker exec -it learninganalyticsapi_app_2 bash
Now go to the root and list files. Slightly different commands than before
cd .. ls
and we get this
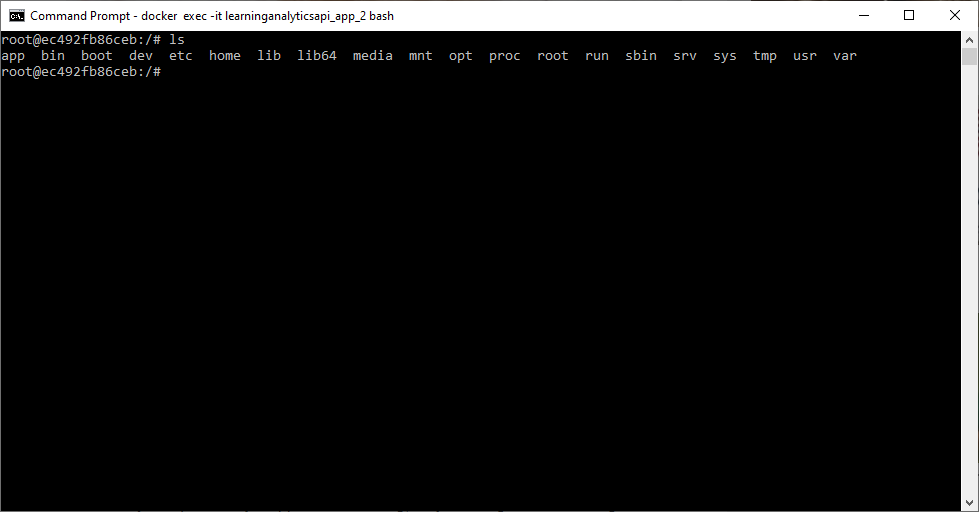
which is the folders at the root of the Linux container.
As before type exit to end the interactive shell and return to the host.
Demo Code
As ever, the source code is on my GitHub site
https://github.com/timbrownls20/Learning-Analytics/tree/master/LearningAnalytics
It’s just an API with a MySQL database. I’m just bringing up the docker container for this demo. The windows Docker file is
and the Linux one is
you could do similar to the above but replace the build and run steps with a docker-compose.yml file. An example is here
https://github.com/timbrownls20/Learning-Analytics/blob/master/LearningAnalytics/docker-compose.yml
which brings up the API container and one for the database. The principle is the same though.